6 Tips to Speed Up Your Laravel Website
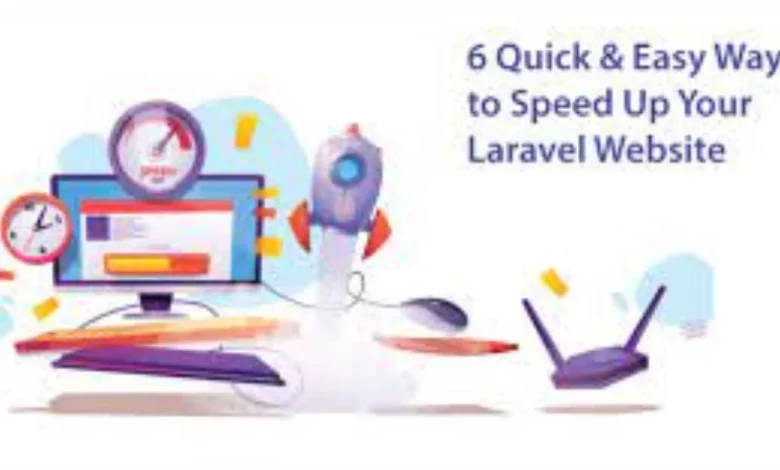
Nearly 1.5M websites run on Laravel.
Even though it keeps production costs and brings a lot of versatility, it can slow your site down if used unprofessionally. This is the reason growing companies with a web application as their product consider hiring a Laravel developer a necessity.
Below we have compiled six quick tips that will give your Laravel website a much-needed boost.
Table of Contents
Only fetch the fields you need in your database queries
Start by decreasing the amount of data transferred between your app and the database. You can do this by highlighting the columns that are needed in your queries through a select clause.
For example, say you have a User model with 10 fields. Now, if you have 10000 users in your database and you’re trying to process them. Your code will appear like this:
The above query will pull 100,000 fields of data.
However, imagine that while processing each user, you only use the id, first_name, and last_name fields. So, out of the 20 fields, 17 of them are redundant for this process. What we can do is define the fields that are needed in the query. Your code will look like:
Consequently, we have decreased the number of fields returned in the query from 100,000 to 15,000. It would reduce the network traffic between Laravel Website app and its database which would to speed up your Laravel site.
This tip might not give you large upgrades on a smaller website or app. However, it can assist in decreasing the load time on apps where performance is paramount.
You will get better improvements if you’re querying tables with BLOB or TEXT fields. These fields hold megabytes of data which increases the query time. If your user model’s table contains either of these, consider defining them.
Implement eager loading if possible
Eager loading is simple with Laravel and prevents you from facing the N+1 issue with your data. The N+1 problem occurs when you make N+1 queries, where N is the number of elements being extracted. To understand check out the example below.
Suppose you have two models, Comment, and Author, with an exclusive relevance within them. Now if you have 100 comments and you want to Laravel Website through everyone and generate the author value.
The code will look like this (without eager loading):
The code would run 101 queries! The first one will fetch all of the comments. The other hundred queries will generate the author’s name in each iteration. This will cause issues and slow down your website.
With eager loading, you can improve the code to this:
The new code looks almost the same. As Laravel Website result, the number of queries ran will reduce from 101 to 2.
Remove unwanted packages
Open the composer.json file and look through all of your dependencies and ask yourself “do I need this?”.
With every new Composer library, you are appending more code that is run unnecessarily. These packages contain service providers that run on unique requests.
For instance, if you add 20 Laravel packages to your app, that’s a minimum of 20 classes being initialized and run on unique requests. Although this isn’t going to affect the performance of small web applications, you’ll be able to see the delta in larger ones.
The solution is to decide whether you need the packages. Perhaps you’re using a package but you’re using a small functionality of it. Write this code and remove this entire package.
This won’t be possible always but, if it is simple and quick then we recommend removing it.
Manage cache properly
Laravel comes with many caching methods by default. These can increase the speed of your website without making any edits to the code.
Route caching
This process turns on the Laravel framework and runs requests that parse the routes file. This needs reading the file, parsing its data, and then presenting it. Laravel has a command that you can use to create a single routes file that is parsed faster:
Also, make sure to run:
This eliminates the cached routes file and registers newer routes. You should add these two commands to deploy the script if you haven’t already.
Config caching
Identical to route caching, Laravel boots up, and each config file is read and parsed. To free them from handling requirements, run the following code which creates one config file:
Like route caching above, you’ll need to run the following code every instance you make edits your .env or config files:
Caching queries and values
Inside the Laravel code of your app, you can cache elements to improve the website’s performance. Consider the following code:
To use caching with it, you should update the query to:
The new code implements the remember() method. It checks whether the cache holds any items with the key users. If it does, the cached value is returned. If it doesn’t, the output of the DB::table(‘users’)->get() code will be the output and will be cached.
Caching data and query results reduce database calls, and runtime, and improve site performance. But, you should remember that you have to remove Laravel Website item from the cache when it’s invalid, sometimes.
Now, imagine that the user’s query is cached. If a new user is created, updated, or deleted, that cached result is invalid and outdated. To fix it, use Laravel model observers to eliminate it from the existing cache.
The next time you try and grab the $users var, a new database query will be running giving you the latest output.
Update to the latest version of PHP
With every new update of PHP, performance becomes better. Kinsta ran tests for various PHP versions on multiple platforms and concluded that PHP 8.1 has the best performance of all.
This tip could be challenging to put into practice as compared to the other tips as you’ll have to audit your current code to ensure an update to the latest version of PHP is safe. On a side note, automating a test suite will give you the data (and confidence) to do this.
Make use of the queues
This tip will take longer than other code-based tips above to show results. Despite this, it will give the biggest performance boost in terms of user experience.
You can cut the runtime down by using the Laravel queues. If any code that is compiled and executed isn’t required for the browser reply, you can “queue it”.
Take a look at the following snippet before queuing:
When the store() method is called it saves the form data, sends an email to a predefined address, and outputs a JSON reply. The problem with this query is that the user needs to wait till the email is sent before they get an update on the browser.
It can cause visitors to churn as it lasts for a few seconds.
To use the queue tactic, update the code:
Here the store() method will store the details in the database, queue the mail, and send an update (JSON response). After the response is sent to the user’s screen, the email is added to the queue for processing.
Now it means that the visitor won’t have to wait several seconds.
We hope that the above tips have helped you. For more, check out Laravel documentation.
VISIT FOR MORE ARTICLE : forbesblog.org